Creating video games is an exciting venture, especially when you can harness the power of JavaScript to bring your ideas to life. In this step-by-step guide, we’ll walk you through the process of building a simple text-based game using JavaScript, with the assistance of ChatGPT for adding interactive elements. By the end of this article, you’ll have a foundational understanding of how to craft your own interactive experiences.
Step 1: Setting Up Your Environment
First things first, let’s set up your development environment. You’ll need a text editor or an Integrated Development Environment (IDE) to write your code. Popular choices include Visual Studio Code, Sublime Text, or Atom.
Additionally, ensure you have a web browser to run your JavaScript code. Any modern browser such as Google Chrome, Mozilla Firefox, or Safari will suffice.
Step 2: Planning Your Game
Before diving into code, it’s essential to plan out your game. Decide on the game’s concept, its mechanics, and the overall flow. For this tutorial, let’s create a simple text-based adventure game where the player navigates through different scenarios by making choices.
Step 3: Writing the HTML Structure
Create an HTML file for your game. This file will serve as the structure of your game and will contain the necessary elements for displaying text and interacting with the player.
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Text Adventure Game</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="game-container">
<h1>Text Adventure Game</h1>
<p id="story">Welcome to the adventure!</p>
<div id="choices">
<button onclick="makeChoice(1)">Choice 1</button>
<button onclick="makeChoice(2)">Choice 2</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Step 4: Styling Your Game (Optional)
You can style your game using CSS to enhance the visual experience. Create a separate styles.css
file and link it to your HTML file.
Step 5: Writing JavaScript Code
Now, it’s time to add interactivity to your game using JavaScript. Create a script.js
file and link it to your HTML file.
javascript
let currentScene = 1;
function displayScene(scene) {
const storyElement = document.getElementById('story');
const choicesElement = document.getElementById('choices');
switch(scene) {
case 1:
storyElement.innerText = "You are standing in front of a mysterious cave. What do you do?";
choicesElement.innerHTML = "<button onclick='makeChoice(2)'>Enter the cave</button>";
break;
case 2:
storyElement.innerText = "You enter the cave and encounter a dragon! What do you do?";
choicesElement.innerHTML = "<button onclick='makeChoice(3)'>Fight the dragon</button><button onclick='makeChoice(4)'>Run away</button>";
break;
// Add more scenes and choices as needed
default:
break;
}
}
function makeChoice(choice) {
switch(currentScene) {
case 1:
if(choice === 2) {
currentScene = 2;
displayScene(currentScene);
}
break;
case 2:
if(choice === 3) {
// Handle fight scene
} else if(choice === 4) {
// Handle run away scene
}
break;
// Add more cases for other scenes
default:
break;
}
}
// Display initial scene
displayScene(currentScene);
Step 6: Adding ChatGPT Integration
To make your game more engaging, you can integrate ChatGPT to provide dynamic responses based on the player’s choices. OpenAI provides an API that you can use to interact with ChatGPT. You’ll need to sign up for access and obtain an API key.
javascript
// Example ChatGPT integration
const apiKey = 'YOUR_API_KEY';
async function getGPTResponse(prompt) {
const response = await fetch('https://api.openai.com/v1/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
},
body: JSON.stringify({
model: 'text-davinci-003',
prompt: prompt,
temperature: 0.7,
max_tokens: 50
})
});
const data = await response.json();
return data.choices[0].text.trim();
}
// Use getGPTResponse() function to get GPT-3 responses based on player choices
Step 7: Testing and Iterating
Test your game thoroughly to ensure everything works as expected. Make adjustments and improvements based on user feedback or your own observations. This iterative process is crucial for refining your game and enhancing the player experience.
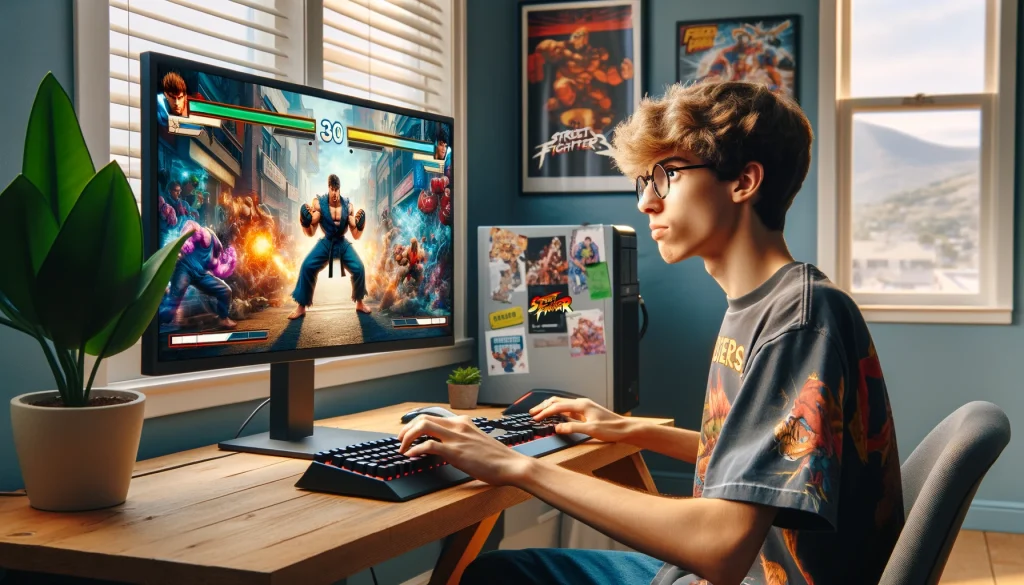
Conclusion
Congratulations! You’ve learned how to create a simple text-based game using JavaScript, with the added interactivity of ChatGPT. From here, you can continue expanding your game with more scenes, choices, and advanced features. Keep experimenting and refining your skills to create even more engaging experiences for your players. Happy coding!